COURSES AND THEIR DETAILS

๐น Introduction to Programming
What is Programming?
Importance of Programming Languages
Choosing the Right Language
๐น C Programming
Basics of C
Variables, Data Types, Operators
Conditional Statements (if-else, switch)
Loops (for, while, do-while)
Functions in C
User-defined & Predefined Functions
Recursion
Arrays and Strings
Pointers and Memory Management
File Handling in C
๐น C++ Programming
Introduction to Object-Oriented Programming
Classes & Objects
Inheritance & Polymorphism
STL (Standard Template Library)
Exception Handling in C++
๐น Java Programming
Basics of Java (JVM, JDK, JRE)
OOP Concepts in Java
Exception Handling
Collections Framework
Multi-threading in Java
File Handling in Java
JDBC (Database Connectivity)
๐น Python Programming
Python Basics (Variables, Data Types, Operators)
Conditional & Looping Statements
Functions and Modules
File Handling
OOP in Python
Python Libraries (NumPy, Pandas, Matplotlib - Basics)
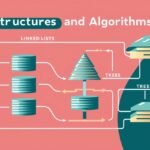
๐น Introduction to Data Structures & Algorithms
Importance of DSA in Problem Solving
Time & Space Complexity (Big-O Notation)
Recursion & Backtracking Basics
๐น Arrays & Strings
Introduction to Arrays & Memory Allocation
Operations on Arrays (Insertion, Deletion, Searching, Sorting)
Two Pointer Technique
Sliding Window Technique
String Manipulation & Pattern Matching (KMP Algorithm, Rabin-Karp)
๐น Linked List
Introduction to Linked List
Types of Linked List (Singly, Doubly, Circular)
Operations (Insertion, Deletion, Reversal)
Floydโs Cycle Detection Algorithm
๐น Stacks and Queues
Stack (Using Arrays & Linked List)
Applications of Stack (Infix to Postfix, Parenthesis Matching)
Queue & Deque Implementation
Priority Queue
๐น Recursion & Backtracking
Recursion Basics & Call Stack
Backtracking Approach (Sudoku Solver, N-Queens Problem)
๐น Sorting & Searching Algorithms
Bubble Sort, Selection Sort, Insertion Sort
Merge Sort, Quick Sort
Binary Search & Variations
๐น Trees & Binary Search Tree (BST)
Introduction to Trees & BST
Traversal Techniques (Inorder, Preorder, Postorder, Level Order)
BST Operations (Insertion, Deletion, Searching)
AVL Tree
๐น Graphs
Graph Representation (Adjacency Matrix & List)
BFS (Breadth First Search) & DFS (Depth First Search)
Shortest Path Algorithms (Dijkstra, Floyd-Warshall)
Minimum Spanning Tree (Kruskal, Primโs Algorithm)
๐น Dynamic Programming (DP)
Introduction to DP & Memoization
Common DP Problems (Knapsack, Longest Common Subsequence, LIS)
Matrix Chain Multiplication
๐น Competitive Programming Practice
Problem Solving on Platforms (LeetCode, CodeChef, HackerRank)
Weekly Coding Challenges
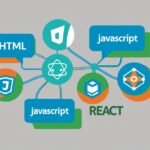
๐น Introduction to Frontend Development
Understanding the Role of a Frontend Developer
Overview of Web Technologies (HTML, CSS, JavaScript)
Introduction to Responsive Web Design
๐น HTML (HyperText Markup Language)
Basics of HTML (Headings, Paragraphs, Lists, Tables)
Forms and Input Handling
HTML5 Features (Audio, Video, Canvas, Semantic Tags)
๐น CSS (Cascading Style Sheets)
Selectors, Colors, and Backgrounds
Box Model & Positioning (Flexbox, Grid)
CSS Animations & Transitions
Media Queries for Responsive Design
๐น JavaScript (JS)
JavaScript Basics (Variables, Functions, Loops, Objects)
DOM Manipulation & Events
ES6+ Features (Arrow Functions, Template Literals, Destructuring)
Asynchronous JavaScript (Callbacks, Promises, Async/Await)
๐น Git & GitHub
Basics of Git (Initializing a Repository, Commits, Branching)
Pushing Projects to GitHub
Collaborating with GitHub
๐น Tailwind CSS
Introduction to Tailwind CSS
Utility-First Approach
Customizing Tailwind & Dark Mode
Responsive Design with Tailwind
๐น React.js (Modern Frontend Framework)
Introduction to React.js & JSX
Functional Components & Props
State Management (useState, useEffect)
React Router for Navigation
API Integration (Fetching Data from APIs)
Context API for State Management
๐น Firebase (Backend for Frontend Devs)
Introduction to Firebase
Firebase Authentication (Google, Email/Password Login)
Firestore Database Basics
Deploying a React App with Firebase
๐น Project Work & Portfolio Building
Building a Responsive Landing Page
Creating a React-based Portfolio Website
Deploying Projects Online (Netlify, Vercel, Firebase Hosting)
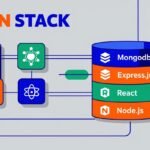
๐น Introduction to Full Stack Development
Overview of MERN Stack
Understanding the Role of Frontend & Backend
Client-Server Architecture
๐น MongoDB (Database)
Introduction to NoSQL & MongoDB
Installing MongoDB & Setting Up a Database
CRUD Operations (Create, Read, Update, Delete)
MongoDB Aggregation & Indexing
Mongoose (Schema & Model)
๐น Express.js (Backend Framework)
Introduction to Express.js
Setting Up a Node.js Server
Creating API Routes (GET, POST, PUT, DELETE)
Middleware & Error Handling
Authentication with JWT (JSON Web Token)
๐น React.js (Frontend Framework)
React Basics (Components, Props, State)
React Router for Navigation
Fetching Data from APIs
Context API & Redux for State Management
React Hooks (useState, useEffect, useContext)
๐น Node.js (Backend Environment)
Introduction to Node.js & NPM
File System & Streams
Building REST APIs with Express
Handling Authentication & Authorization
๐น Connecting Frontend with Backend
Fetching Data from Express API in React
Implementing User Authentication (JWT + React Context)
Protecting Routes in React
๐น Deployment & Project Work
Deploying Backend on Render / Railway / Vercel
Deploying Frontend on Netlify / Vercel
Connecting Backend with MongoDB Atlas
Full-Stack MERN Project:
User Authentication System
Blog Website with CRUD Features
E-commerce Platform (Basic)
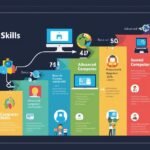
๐น Basic Computer Skills
Understanding Computer Hardware & Software
Operating Systems (Windows, macOS, Linux)
File Management (Creating, Renaming, Deleting Files/Folders)
Installing & Uninstalling Software
๐น Internet & Networking Basics
Understanding the Internet & Web Browsers
Basics of Networking (LAN, WAN, Wi-Fi, IP Address)
Safe Browsing & Cybersecurity Basics
๐น Microsoft Office / Google Workspace
MS Word / Google Docs (Document Formatting, Templates)
MS Excel / Google Sheets (Basic Formulas, Charts, Pivot Tables)
MS PowerPoint / Google Slides (Creating Presentations)
๐น Email & Online Communication
Creating & Managing Emails (Gmail, Outlook)
Writing Professional Emails
Online Meetings & Collaboration (Google Meet, Zoom)
๐น Typing & Shortcuts
Improving Typing Speed & Accuracy
Keyboard Shortcuts for Windows & macOS
๐น Intermediate Level Topics
๐น Advanced Excel & Data Handling
Formulas & Functions (VLOOKUP, HLOOKUP, IF, SUMIF)
Data Sorting, Filtering & Validation
Conditional Formatting
Creating Reports & Dashboards
๐น Introduction to Programming
Basics of Programming (C, Python)
Writing & Executing Simple Programs
Logical Thinking & Problem Solving
๐น Computer Troubleshooting
Common Software & Hardware Issues
Antivirus & System Security
Basic Troubleshooting & System Maintenance
๐น Advanced Computer Skills
๐น Operating Systems & Command Line
Windows Command Prompt & PowerShell
Linux Basics (Terminal Commands, File Permissions)
๐น Introduction to Cloud Computing
Google Drive, Dropbox, OneDrive
Cloud-Based Collaboration & Storage
๐น Cybersecurity & Ethical Hacking Basics
Understanding Phishing, Malware, Ransomware
Password Management & Two-Factor Authentication
Basic Network Security
๐น Resume Building & Job Preparation
How to Create a Strong Resume
Cover Letter Writing
Online Job Portals & Profile Optimization (LinkedIn, Naukri)
๐น Final Project & Certification
Hands-on Project:
Create an Automated Excel Report
Build a Resume Using MS Word
Prepare a Presentation on Cybersecurity

๐น Introduction to Placement Process
Understanding Campus & Off-Campus Placements
Different Hiring Processes (Walk-ins, Referral, Direct Applications)
Importance of Resume & LinkedIn Profile
๐น Resume & Cover Letter Building
Creating an ATS-Friendly Resume
Writing an Impactful Cover Letter
Customizing Resume for Different Job Roles
Doโs & Donโts in Resume Writing
๐น Aptitude & Logical Reasoning
Quantitative Aptitude (Profit & Loss, Time & Work, Probability)
Logical Reasoning (Puzzles, Coding-Decoding, Data Interpretation)
Verbal Ability (Reading Comprehension, Grammar, Sentence Correction)
๐น Technical Interview Preparation
๐ธ Programming & Data Structures
Language-Specific Preparation (C/C++, Java, Python)
Important DSA Topics (Arrays, Strings, Recursion, Linked Lists)
Solving Coding Challenges (LeetCode, CodeChef, HackerRank)
๐ธ Core CS Subjects
Operating Systems (Process Management, Memory Management)
DBMS (Normalization, Indexing, SQL Queries)
Computer Networks (TCP/IP, HTTP, Routing Protocols)
๐ธ System Design & OOP Concepts
Understanding Low-Level & High-Level Design
OOPS (Encapsulation, Inheritance, Polymorphism)
Design Patterns & Real-Life Scenarios
๐น HR & Behavioral Interview Preparation
Most Common HR Interview Questions
STAR Method for Answering Behavioral Questions
Negotiation & Salary Discussion Strategies
Handling Stress Interviews
๐น Group Discussion & Communication Skills
Techniques for Effective Communication
How to Speak Confidently in Interviews
Group Discussion Doโs & Donโts
๐น Final Mock Interviews & Assessments
Live Mock Interviews (Technical + HR)
Feedback & Improvement Suggestions
Personalized Career Guidance
๐น Final Project & Certification
Creating a Portfolio Website
Writing a Tech Blog on LinkedIn
Final Resume & LinkedIn Profile Submission

๐น Introduction to Data Analytics
What is Data Analytics?
Importance of Data in Decision Making
Types of Data Analytics (Descriptive, Diagnostic, Predictive, Prescriptive)
Career Opportunities in Data Analytics
๐น Excel for Data Analysis
Data Cleaning & Formatting
Conditional Formatting & Data Validation
Pivot Tables & Charts
Advanced Excel Formulas (VLOOKUP, HLOOKUP, INDEX-MATCH)
๐น SQL for Data Analysis
Introduction to Databases & SQL
Writing SQL Queries (SELECT, WHERE, GROUP BY, HAVING)
Joins & Subqueries
Aggregate Functions (SUM, AVG, COUNT)
Optimizing SQL Queries
๐น Python for Data Analytics
Introduction to Python & Jupyter Notebook
Data Types, Lists, Tuples, Dictionaries
Pandas for Data Manipulation
NumPy for Numerical Analysis
Data Visualization with Matplotlib & Seaborn
๐น Data Cleaning & Preprocessing
Handling Missing Values
Data Transformation & Encoding
Outlier Detection & Handling
Feature Scaling & Normalization
๐น Data Visualization & Business Insights
Creating Interactive Dashboards
Data Storytelling with Power BI / Tableau
Creating Reports & Business Insights
๐น Exploratory Data Analysis (EDA)
Identifying Trends & Patterns in Data
Correlation & Regression Analysis
Using Statistical Methods for Decision Making
๐น Machine Learning Basics (Optional)
Introduction to Machine Learning
Supervised vs Unsupervised Learning
Implementing Simple ML Models (Linear Regression, Decision Trees)
๐น Final Project & Certification
Real-World Data Analysis Project
Creating a Data Report & Dashboard
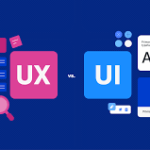
๐น Introduction to UI/UX Design
What is UI/UX? Difference Between UI & UX
Importance of UI/UX in Modern Applications
Career Opportunities in UI/UX
๐น UX (User Experience) Fundamentals
Understanding User Psychology & Behavior
UX Research Methods (User Interviews, Surveys)
Creating User Personas & User Journey Maps
Competitor Analysis
๐น Wireframing & Prototyping
Introduction to Wireframes
Low-Fidelity vs High-Fidelity Wireframes
Tools for Wireframing (Figma, Adobe XD, Balsamiq)
Creating Clickable Prototypes
๐น UI (User Interface) Design Principles
Color Theory & Typography
Visual Hierarchy & Layout Design
Consistency & Design Patterns
Responsive & Adaptive Design
๐น Design Tools & Software
Figma (Complete Hands-on)
Adobe XD / Sketch Basics
Using Plugins & Components in Figma
๐น Interaction & Animation
Microinteractions & Motion UI
Creating Smooth UI Animations
Usability Testing for Interactions
๐น UX Writing & Content Strategy
Writing Effective Microcopy
CTA (Call-to-Action) Best Practices
Content Strategy for Websites & Apps
OUR WEBINAR
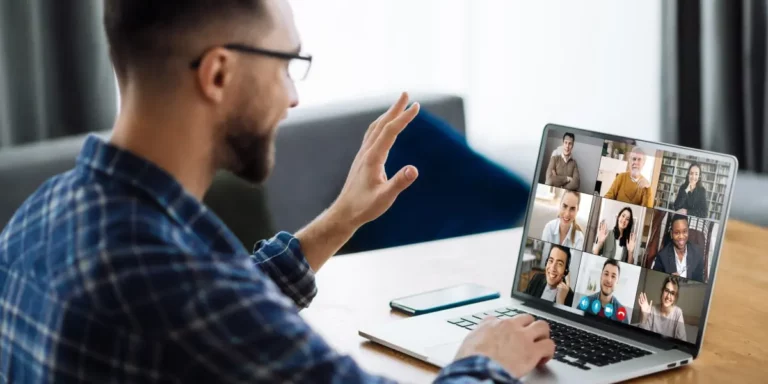
Coding Courses & Bootcamps
- Beginner to Advanced Courses (HTML, CSS, JavaScript, Python, etc.)
- Full-Stack Web Development (MERN, LAMP, etc.)
- Mobile App Development (React Native, Flutter, Swift, Kotlin)
- Data Science & Machine Learning
- Game Development (Unity, Unreal Engine)
- Cybersecurity & Ethical Hacking

One-on-One Coaching & Mentorship
- Personalized coding lessons
- Career guidance & roadmap planning
- Code reviews & debugging help
- Project-based learning & portfolio building

Exam & Interview Preparation
- Coding interview prep (LeetCode, system design, DSA)
- Technical resume & portfolio review
- Mock interviews (FAANG-style)
- College entrance test prep (CS-related exams)